static class Program
{
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
using (var chartForm = new ChartForm())
{
FillData(chartForm.Chart);
chartForm.Show();
//save
chartForm.Chart.SaveImage(@"Chart.png", ChartImageFormat.Png);
chartForm.Close();
}
}
public static void FillData(Chart chart)
{
chart.Titles[0].Text = "Price/Volume Chart";
chart.Series[0].Name = "Price";
chart.Series[1].Name = "Volume";
var random = new Random();
for (int i = 0; i < 10; i++)
{
var date = DateTime.Today.AddDays(i);
chart.Series[0].Points.Add(new DataPoint(date.ToOADate(), random.NextDouble() * 1e3));
chart.Series[1].Points.Add(new DataPoint(date.ToOADate(), random.Next() / 1e3));
}
}
}
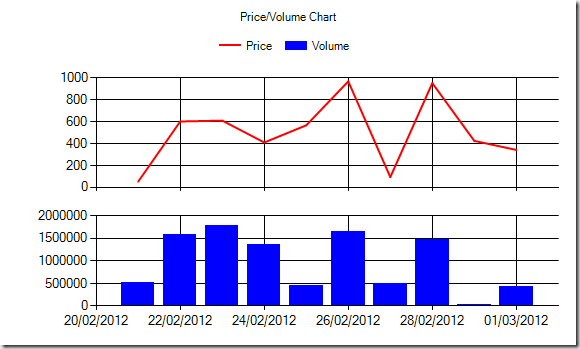
//Designer Code
private void InitializeComponent()
{
System.Windows.Forms.DataVisualization.Charting.ChartArea chartArea1 = new System.Windows.Forms.DataVisualization.Charting.ChartArea();
System.Windows.Forms.DataVisualization.Charting.ChartArea chartArea2 = new System.Windows.Forms.DataVisualization.Charting.ChartArea();
System.Windows.Forms.DataVisualization.Charting.Legend legend1 = new System.Windows.Forms.DataVisualization.Charting.Legend();
System.Windows.Forms.DataVisualization.Charting.Series series1 = new System.Windows.Forms.DataVisualization.Charting.Series();
System.Windows.Forms.DataVisualization.Charting.Series series2 = new System.Windows.Forms.DataVisualization.Charting.Series();
System.Windows.Forms.DataVisualization.Charting.Title title1 = new System.Windows.Forms.DataVisualization.Charting.Title();
this.chart1 = new System.Windows.Forms.DataVisualization.Charting.Chart();
((System.ComponentModel.ISupportInitialize)(this.chart1)).BeginInit();
this.SuspendLayout();
//
// chart1
//
chartArea1.AxisX.LabelStyle.Enabled = false;
chartArea1.Name = "ChartArea1";
chartArea2.AlignWithChartArea = "ChartArea1";
chartArea2.Name = "ChartArea2";
this.chart1.ChartAreas.Add(chartArea1);
this.chart1.ChartAreas.Add(chartArea2);
this.chart1.Dock = System.Windows.Forms.DockStyle.Fill;
legend1.Alignment = System.Drawing.StringAlignment.Center;
legend1.Docking = System.Windows.Forms.DataVisualization.Charting.Docking.Top;
legend1.Name = "Legend1";
this.chart1.Legends.Add(legend1);
this.chart1.Location = new System.Drawing.Point(0, 0);
this.chart1.Name = "chart1";
series1.BorderWidth = 2;
series1.ChartArea = "ChartArea1";
series1.ChartType = System.Windows.Forms.DataVisualization.Charting.SeriesChartType.Line;
series1.Color = System.Drawing.Color.Red;
series1.Legend = "Legend1";
series1.Name = "Series1";
series1.XValueType = System.Windows.Forms.DataVisualization.Charting.ChartValueType.Date;
series2.ChartArea = "ChartArea2";
series2.Color = System.Drawing.Color.Blue;
series2.Legend = "Legend1";
series2.Name = "Series2";
series2.XValueType = System.Windows.Forms.DataVisualization.Charting.ChartValueType.Date;
this.chart1.Series.Add(series1);
this.chart1.Series.Add(series2);
this.chart1.Size = new System.Drawing.Size(466, 248);
this.chart1.TabIndex = 0;
this.chart1.Text = "chart1";
title1.Name = "Title1";
title1.Text = "<TITLE>";
this.chart1.Titles.Add(title1);
//
// ChartControl
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.Controls.Add(this.chart1);
this.Name = "ChartControl";
this.Size = new System.Drawing.Size(466, 248);
((System.ComponentModel.ISupportInitialize)(this.chart1)).EndInit();
this.ResumeLayout(false);
}
Create Chart in C#
ReplyDelete